127 lines
3.4 KiB
Markdown
127 lines
3.4 KiB
Markdown
# Leetcode Flood-Fill
|
|
|
|
#### 2022-07-15 09:01
|
|
|
|
> ##### Algorithms:
|
|
> #algorithm #BFS
|
|
> ##### Data structures:
|
|
> #DS
|
|
> ##### Difficulty:
|
|
> #coding_problem #difficulty-easy
|
|
> ##### Additional tags:
|
|
> #leetcode
|
|
> ##### Revisions:
|
|
> N/A
|
|
|
|
##### Related topics:
|
|
##### Links:
|
|
- [Link to problem](https://leetcode.com/problems/flood-fill/)
|
|
___
|
|
### Problem
|
|
|
|
An image is represented by an `m x n` integer grid `image` where `image[i][j]` represents the pixel value of the image.
|
|
|
|
You are also given three integers `sr`, `sc`, and `color`. You should perform a **flood fill** on the image starting from the pixel `image[sr][sc]`.
|
|
|
|
To perform a **flood fill**, consider the starting pixel, plus any pixels connected **4-directionally** to the starting pixel of the same color as the starting pixel, plus any pixels connected **4-directionally** to those pixels (also with the same color), and so on. Replace the color of all of the aforementioned pixels with `color`.
|
|
|
|
Return _the modified image after performing the flood fill_.
|
|
|
|
#### Examples
|
|
|
|
**Example 1:**
|
|
|
|
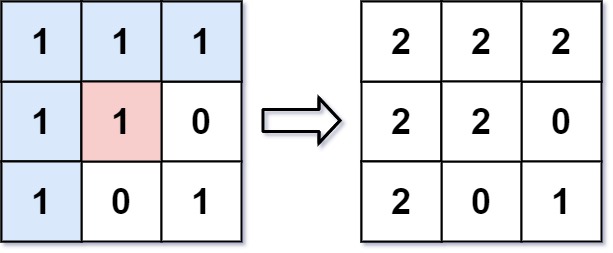
|
|
|
|
```
|
|
**Input:** image = [[1,1,1],[1,1,0],[1,0,1]], sr = 1, sc = 1, color = 2
|
|
**Output:** [[2,2,2],[2,2,0],[2,0,1]]
|
|
**Explanation:** From the center of the image with position (sr, sc) = (1, 1) (i.e., the red pixel), all pixels connected by a path of the same color as the starting pixel (i.e., the blue pixels) are colored with the new color.
|
|
Note the bottom corner is not colored 2, because it is not 4-directionally connected to the starting pixel.
|
|
```
|
|
|
|
**Example 2:**
|
|
|
|
```
|
|
**Input:** image = [[0,0,0],[0,0,0]], sr = 0, sc = 0, color = 0
|
|
**Output:** [[0,0,0],[0,0,0]]
|
|
**Explanation:** The starting pixel is already colored 0, so no changes are made to the image.
|
|
```
|
|
|
|
#### Constraints
|
|
|
|
- `m == image.length`
|
|
- `n == image[i].length`
|
|
- `1 <= m, n <= 50`
|
|
- `0 <= image[i][j], color < 216`
|
|
- `0 <= sr < m`
|
|
- `0 <= sc < n`
|
|
|
|
### Thoughts
|
|
|
|
> [!summary]
|
|
> This is a search problem, can be solved using DFS or BFS
|
|
|
|
This one can be optimized.
|
|
|
|
Initially, I wanted to use a hash map to record cells that are visited,
|
|
but the this takes up extra space.
|
|
|
|
Then I found out that I can use colors:
|
|
- if image[r][c] == color, this means
|
|
- the cell is no need to correct(color == origcolor),
|
|
- or this has been corrected
|
|
so I don't have to go over again.
|
|
|
|
There are checks in the loop:
|
|
- check the color is not visited, as shown above
|
|
- check the coord is not OOB
|
|
- check the cell is equal to origColor, to only fill same origColor.
|
|
|
|
#TODO: Write in DFS
|
|
|
|
### Solution
|
|
|
|
```cpp
|
|
class Solution {
|
|
public:
|
|
vector<vector<int>> floodFill(vector<vector<int>> &image, int sr, int sc,
|
|
int color) {
|
|
int origColor = image[sr][sc];
|
|
queue<pair<int, int>> todo;
|
|
int m = image.size();
|
|
int n = image[0].size();
|
|
|
|
todo.push({sr, sc});
|
|
int r, c;
|
|
|
|
while (!todo.empty()) {
|
|
r = todo.front().first;
|
|
c = todo.front().second;
|
|
todo.pop();
|
|
|
|
if (image[r][c] != origColor || image[r][c] == color) {
|
|
// already colored
|
|
continue;
|
|
}
|
|
|
|
image[r][c] = color;
|
|
|
|
if (r > 0) {
|
|
todo.push({r - 1, c});
|
|
}
|
|
if (r < m - 1) {
|
|
todo.push({r + 1, c});
|
|
}
|
|
if (c > 0) {
|
|
todo.push({r, c - 1});
|
|
}
|
|
if (c < n - 1) {
|
|
todo.push({r, c + 1});
|
|
}
|
|
}
|
|
|
|
return image;
|
|
}
|
|
};
|
|
``` |