135 lines
2.7 KiB
Markdown
135 lines
2.7 KiB
Markdown
# Leetcode Maximum-Depth-Of-Binary-Tree
|
||
|
||
#### 2022-07-05 09:25
|
||
|
||
> ##### Algorithms:
|
||
> #algorithm #BFS
|
||
> ##### Data structures:
|
||
> #DS #binary_tree
|
||
> ##### Difficulty:
|
||
> #coding_problem #difficulty-easy
|
||
> ##### Additional tags:
|
||
> #leetcode
|
||
> ##### Revisions:
|
||
> N/A
|
||
|
||
##### Related topics:
|
||
```expander
|
||
tag:#BFS
|
||
```
|
||
|
||
- [[Leetcode Binary-Tree-Level-Order-Traversal]]
|
||
- [[Leetcode Search-In-a-Binary-Tree]]
|
||
|
||
|
||
##### Links:
|
||
- [Link to problem](https://leetcode.com/problems/maximum-depth-of-binary-tree/)
|
||
___
|
||
### Problem
|
||
Given the `root` of a binary tree, return _its maximum depth_.
|
||
|
||
A binary tree's **maximum depth** is the number of nodes along the longest path from the root node down to the farthest leaf node.
|
||
|
||
#### Examples
|
||
|
||
**Example 1:**
|
||
|
||
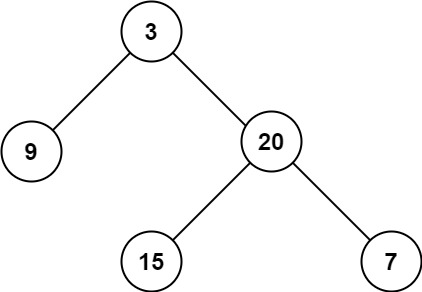
|
||
|
||
**Input:** root = [3,9,20,null,null,15,7]
|
||
**Output:** 3
|
||
|
||
**Example 2:**
|
||
|
||
**Input:** root = [1,null,2]
|
||
**Output:** 2
|
||
|
||
#### Constraints
|
||
|
||
- The number of nodes in the tree is in the range `[0, 104]`.
|
||
- `-100 <= Node.val <= 100`
|
||
|
||
### Thoughts
|
||
|
||
> [!summary]
|
||
> This problem can be solved by #BFS or #DFS
|
||
|
||
BFS way:
|
||
Simply log the level in each while iteration.
|
||
|
||
DFS way: (Popular)
|
||
Use recursion:
|
||
- Base Case:
|
||
- root == nullptr: return 0;
|
||
- maxDepth(root) = max(maxDepth(root->left), maxDepth(root->right))
|
||
|
||
### Solution
|
||
|
||
DFS Recursion:
|
||
```cpp
|
||
/**
|
||
* Definition for a binary tree node.
|
||
* struct TreeNode {
|
||
* int val;
|
||
* TreeNode *left;
|
||
* TreeNode *right;
|
||
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
|
||
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
|
||
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left),
|
||
* right(right) {}
|
||
* };
|
||
*/
|
||
class Solution {
|
||
public:
|
||
int maxDepth(TreeNode *root) {
|
||
// DFS
|
||
if (!root) {
|
||
return 0;
|
||
}
|
||
|
||
return max(maxDepth(root->left), maxDepth(root->right)) + 1;
|
||
}
|
||
};
|
||
```
|
||
|
||
BFS:
|
||
```cpp
|
||
/**
|
||
* Definition for a binary tree node.
|
||
* struct TreeNode {
|
||
* int val;
|
||
* TreeNode *left;
|
||
* TreeNode *right;
|
||
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
|
||
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
|
||
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left),
|
||
* right(right) {}
|
||
* };
|
||
*/
|
||
class Solution {
|
||
public:
|
||
int maxDepth(TreeNode *root) {
|
||
// BFS
|
||
int levels = 0;
|
||
queue<TreeNode *> pending;
|
||
TreeNode *ptr = root;
|
||
if (ptr)
|
||
pending.push(ptr);
|
||
|
||
while (!pending.empty()) {
|
||
levels++;
|
||
for (int i = 0, size = pending.size(); i < size; i++) {
|
||
ptr = pending.front();
|
||
pending.pop();
|
||
|
||
if (ptr->left)
|
||
pending.push(ptr->left);
|
||
if (ptr->right)
|
||
pending.push(ptr->right);
|
||
}
|
||
}
|
||
|
||
return levels;
|
||
}
|
||
};
|
||
``` |