vault backup: 2022-06-15 22:12:54
This commit is contained in:
parent
10a707bea2
commit
74ebcda764
|
@ -30,6 +30,7 @@ tag:#leetcode
|
||||||
- [[Leetcode Merge-Two-Sorted-Lists]]
|
- [[Leetcode Merge-Two-Sorted-Lists]]
|
||||||
- [[Leetcode Pascal's-Triangle]]
|
- [[Leetcode Pascal's-Triangle]]
|
||||||
- [[Leetcode Ransom-Note]]
|
- [[Leetcode Ransom-Note]]
|
||||||
|
- [[Leetcode Remove-Linked-List-Elements]]
|
||||||
- [[Leetcode Reshape-The-Matrix]]
|
- [[Leetcode Reshape-The-Matrix]]
|
||||||
- [[Leetcode Search-a-2D-Matrix]]
|
- [[Leetcode Search-a-2D-Matrix]]
|
||||||
- [[Leetcode Two-Sum]]
|
- [[Leetcode Two-Sum]]
|
||||||
|
@ -66,6 +67,7 @@ tag:#DS tag:#coding_problem -tag:#template_remove_me
|
||||||
- [[Leetcode Merge-Two-Sorted-Lists]]
|
- [[Leetcode Merge-Two-Sorted-Lists]]
|
||||||
- [[Leetcode Pascal's-Triangle]]
|
- [[Leetcode Pascal's-Triangle]]
|
||||||
- [[Leetcode Ransom-Note]]
|
- [[Leetcode Ransom-Note]]
|
||||||
|
- [[Leetcode Remove-Linked-List-Elements]]
|
||||||
- [[Leetcode Reshape-The-Matrix]]
|
- [[Leetcode Reshape-The-Matrix]]
|
||||||
- [[Leetcode Search-a-2D-Matrix]]
|
- [[Leetcode Search-a-2D-Matrix]]
|
||||||
- [[Leetcode Two-Sum]]
|
- [[Leetcode Two-Sum]]
|
||||||
|
@ -80,6 +82,7 @@ tag:#DS tag:#CS_analysis -tag:#template_remove_me
|
||||||
- [[$filename]]
|
- [[$filename]]
|
||||||
```
|
```
|
||||||
|
|
||||||
|
- [[Binary Search Algorithm.sync-conflict-20220615-121016-T44CT3O]]
|
||||||
- [[cpp_Range_based_for_loop]]
|
- [[cpp_Range_based_for_loop]]
|
||||||
- [[cpp_std_multiset]]
|
- [[cpp_std_multiset]]
|
||||||
- [[cpp_std_unordered_map]]
|
- [[cpp_std_unordered_map]]
|
||||||
|
@ -118,11 +121,5 @@ tag:#algorithm tag:#CS_analysis -tag:#template_remove_me
|
||||||
- [[$filename]]
|
- [[$filename]]
|
||||||
```
|
```
|
||||||
|
|
||||||
- [[Binary Search Algorithm]]
|
|
||||||
- [[cpp_std_sort]]
|
|
||||||
- [[Floyd's Cycle Finding Algorithm]]
|
|
||||||
- [[Kadane's Algorithm]]
|
|
||||||
- [[Two pointers approach]]
|
|
||||||
|
|
||||||
|
|
||||||
|
|
|
@ -3,33 +3,145 @@
|
||||||
#### 2022-06-15 21:50
|
#### 2022-06-15 21:50
|
||||||
|
|
||||||
---
|
---
|
||||||
##### Algorithms:
|
|
||||||
#algorithm
|
|
||||||
##### Data structures:
|
##### Data structures:
|
||||||
#DS
|
#DS #linked_list
|
||||||
##### Difficulty:
|
##### Difficulty:
|
||||||
#<CHANGE_ME> #coding_problem #difficulty-easy
|
#leetcode #coding_problem #difficulty-easy
|
||||||
##### Related topics:
|
##### Related topics:
|
||||||
```expander
|
```expander
|
||||||
tag:#<INSERT_TAG_HERE>
|
tag:#linked_list
|
||||||
```
|
```
|
||||||
|
|
||||||
|
- [[Floyd's Cycle Finding Algorithm]]
|
||||||
|
- [[Leetcode Linked-List-Cycle]]
|
||||||
|
- [[Leetcode Merge-Two-Sorted-Lists]]
|
||||||
|
- [[Two pointers approach]]
|
||||||
|
|
||||||
|
|
||||||
##### Links:
|
##### Links:
|
||||||
- [Link to problem]()
|
- [Link to problem](https://leetcode.com/problems/remove-linked-list-elements/)
|
||||||
|
- [Additional Solutions](https://leetcode.com/problems/remove-linked-list-elements/discuss/722528/C++-2-solutions:-With-single-pointer-+-With-double-pointers-(Easy-to-understand)/1390186)
|
||||||
___
|
___
|
||||||
### Problem
|
### Problem
|
||||||
|
Given the `head` of a linked list and an integer `val`, remove all the nodes of the linked list that has `Node.val == val`, and return _the new head_.
|
||||||
|
|
||||||
#### Examples
|
#### Examples
|
||||||
|
**Example 1:**
|
||||||
|
|
||||||
|
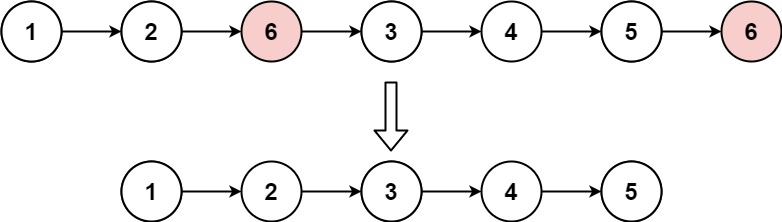
|
||||||
|
|
||||||
```markdown
|
```markdown
|
||||||
|
**Input:** head = [1,2,6,3,4,5,6], val = 6
|
||||||
|
**Output:** [1,2,3,4,5]
|
||||||
```
|
```
|
||||||
|
|
||||||
|
**Example 2:**
|
||||||
|
|
||||||
|
```markdown
|
||||||
|
**Input:** head = [], val = 1
|
||||||
|
**Output:** []
|
||||||
|
```
|
||||||
|
|
||||||
|
**Example 3:**
|
||||||
|
|
||||||
|
```markdown
|
||||||
|
**Input:** head = [7,7,7,7], val = 7
|
||||||
|
**Output:** []
|
||||||
|
```
|
||||||
#### Constraints
|
#### Constraints
|
||||||
|
- The number of nodes in the list is in the range `[0, 104]`.
|
||||||
|
- `1 <= Node.val <= 50`
|
||||||
|
- `0 <= val <= 50`
|
||||||
|
|
||||||
### Thoughts
|
### Thoughts
|
||||||
|
|
||||||
> [!summary]
|
Simple linked list operations, but remember to check for special cases:
|
||||||
> This is a #template_remove_me
|
- The pointer is null
|
||||||
|
|
||||||
### Solution
|
### Solution
|
||||||
|
|
||||||
|
Two pointers, O(n)
|
||||||
|
```cpp
|
||||||
|
/**
|
||||||
|
* Definition for singly-linked list.
|
||||||
|
* struct ListNode {
|
||||||
|
* int val;
|
||||||
|
* ListNode *next;
|
||||||
|
* ListNode() : val(0), next(nullptr) {}
|
||||||
|
* ListNode(int x) : val(x), next(nullptr) {}
|
||||||
|
* ListNode(int x, ListNode *next) : val(x), next(next) {}
|
||||||
|
* };
|
||||||
|
*/
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
ListNode *removeElements(ListNode *head, int val) {
|
||||||
|
// O(n)
|
||||||
|
while (head != NULL && head->val == val) {
|
||||||
|
head = head->next;
|
||||||
|
}
|
||||||
|
|
||||||
|
ListNode *before = NULL;
|
||||||
|
ListNode *ptr = head;
|
||||||
|
ListNode *tmp;
|
||||||
|
|
||||||
|
while (ptr != NULL) {
|
||||||
|
if (ptr->val == val) {
|
||||||
|
if (before != NULL) {
|
||||||
|
before->next = ptr->next;
|
||||||
|
}
|
||||||
|
|
||||||
|
// delete ptr and change ptr to ptr->next
|
||||||
|
tmp = ptr->next;
|
||||||
|
delete ptr;
|
||||||
|
ptr = tmp;
|
||||||
|
} else {
|
||||||
|
before = ptr;
|
||||||
|
ptr = ptr->next;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
return head;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
|
||||||
|
These two are taken from discussions, and they are **not** memory safe.
|
||||||
|
Recursive solution from the same guy:
|
||||||
|
```cpp
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
ListNode *removeElements(ListNode *head, int val) {
|
||||||
|
// Base situation
|
||||||
|
if (head == NULL)
|
||||||
|
return NULL;
|
||||||
|
// Change head->next by it's val (if no val found, will not be changed)
|
||||||
|
head->next = removeElements(head->next, val);
|
||||||
|
// Return head or head->next, depending on the val.
|
||||||
|
// If matched val, return its next, effectively deleting the node.
|
||||||
|
return (head->val == val) ? head->next : head;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
|
||||||
|
One pointer from [Discussions](https://leetcode.com/problems/remove-linked-list-elements/discuss/722528/C++-2-solutions:-With-single-pointer-+-With-double-pointers-(Easy-to-understand)/1390186)
|
||||||
|
```cpp
|
||||||
|
class Solution {
|
||||||
|
public:
|
||||||
|
ListNode *removeElements(ListNode *head, int val) {
|
||||||
|
while (head != NULL && head->val == val)
|
||||||
|
head = head->next;
|
||||||
|
|
||||||
|
// He checked NULL here, so he doesn't have to check in while loop
|
||||||
|
if (head == NULL)
|
||||||
|
return head;
|
||||||
|
|
||||||
|
ListNode *res = head;
|
||||||
|
while (head->next != NULL) {
|
||||||
|
if (head->next->val == val)
|
||||||
|
head->next = head->next->next;
|
||||||
|
else
|
||||||
|
head = head->next;
|
||||||
|
}
|
||||||
|
return res;
|
||||||
|
}
|
||||||
|
};
|
||||||
|
```
|
||||||
|
|
||||||
|
|
35
CS notes/pages/Leetcode Reverse-Linked-List.md
Normal file
35
CS notes/pages/Leetcode Reverse-Linked-List.md
Normal file
|
@ -0,0 +1,35 @@
|
||||||
|
# Leetcode Reverse-Linked-List
|
||||||
|
|
||||||
|
#### 2022-06-15 22:07
|
||||||
|
|
||||||
|
---
|
||||||
|
##### Algorithms:
|
||||||
|
#algorithm #recursion
|
||||||
|
##### Data structures:
|
||||||
|
#DS #linked_list
|
||||||
|
##### Difficulty:
|
||||||
|
#leetcode #coding_problem #difficulty-easy
|
||||||
|
##### Related topics:
|
||||||
|
```expander
|
||||||
|
tag:#linked_list
|
||||||
|
```
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
##### Links:
|
||||||
|
- [Link to problem]()
|
||||||
|
___
|
||||||
|
### Problem
|
||||||
|
|
||||||
|
#### Examples
|
||||||
|
```markdown
|
||||||
|
```
|
||||||
|
|
||||||
|
#### Constraints
|
||||||
|
|
||||||
|
### Thoughts
|
||||||
|
|
||||||
|
> [!summary]
|
||||||
|
> This is a #template_remove_me
|
||||||
|
|
||||||
|
### Solution
|
Loading…
Reference in a new issue