2022-07-04 15:01:29 +08:00
|
|
|
# Leetcode Binary-Tree-Preorder-Traversal
|
|
|
|
|
|
|
|
#### 2022-07-04 14:51
|
|
|
|
|
|
|
|
> ##### Algorithms:
|
2022-07-04 15:50:54 +08:00
|
|
|
> #algorithm #DFS #DFS_preorder #Morris_traversal
|
2022-07-04 15:01:29 +08:00
|
|
|
> ##### Data structures:
|
|
|
|
> #DS #binary_tree
|
|
|
|
> ##### Difficulty:
|
|
|
|
> #coding_problem #difficulty-easy
|
|
|
|
> ##### Additional tags:
|
2022-07-04 15:50:54 +08:00
|
|
|
> #leetcode #CS_list_need_understanding
|
2022-07-04 15:01:29 +08:00
|
|
|
> ##### Revisions:
|
|
|
|
> N/A
|
|
|
|
|
|
|
|
##### Related topics:
|
|
|
|
```expander
|
2022-07-04 15:50:54 +08:00
|
|
|
tag:#DFS
|
2022-07-04 15:01:29 +08:00
|
|
|
```
|
|
|
|
|
2022-07-04 15:50:54 +08:00
|
|
|
- [[Leetcode Binary-Tree-Inorder-Traversal]]
|
2022-07-05 09:08:49 +08:00
|
|
|
- [[Leetcode Binary-Tree-Postorder-Traversal]]
|
2022-07-08 11:29:47 +08:00
|
|
|
- [[Leetcode Insert-Into-a-Binary-Search-Tree]]
|
2022-07-07 08:16:24 +08:00
|
|
|
- [[Leetcode Invert-Binary-Tree]]
|
2022-07-05 10:48:41 +08:00
|
|
|
- [[Leetcode Maximum-Depth-Of-Binary-Tree]]
|
2022-07-07 08:16:24 +08:00
|
|
|
- [[Leetcode Path-Sum]]
|
|
|
|
- [[Leetcode Search-In-a-Binary-Tree]]
|
2022-07-05 10:48:41 +08:00
|
|
|
- [[Leetcode Symmetric-Tree]]
|
2022-07-08 11:29:47 +08:00
|
|
|
- [[Leetcode Validate-Binary-Search-Tree]]
|
2022-07-04 15:01:29 +08:00
|
|
|
|
|
|
|
|
|
|
|
##### Links:
|
|
|
|
- [Link to problem](https://leetcode.com/problems/binary-tree-preorder-traversal/)
|
|
|
|
___
|
|
|
|
### Problem
|
|
|
|
Given the `root` of a binary tree, return _the preorder traversal of its nodes' values_.
|
|
|
|
|
|
|
|
#### Examples
|
|
|
|
**Example 1:**
|
|
|
|
|
|
|
|
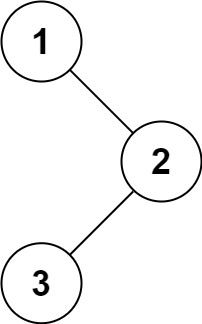
|
|
|
|
|
|
|
|
**Input:** root = [1,null,2,3]
|
|
|
|
**Output:** [1,2,3]
|
|
|
|
|
|
|
|
**Example 2:**
|
|
|
|
|
|
|
|
**Input:** root = []
|
|
|
|
**Output:** []
|
|
|
|
|
|
|
|
**Example 3:**
|
|
|
|
|
|
|
|
**Input:** root = [1]
|
|
|
|
**Output:** [1]
|
|
|
|
#### Constraints
|
|
|
|
- The number of nodes in the tree is in the range `[0, 100]`.
|
|
|
|
- `-100 <= Node.val <= 100`
|
|
|
|
### Thoughts
|
|
|
|
|
|
|
|
> [!summary]
|
|
|
|
> This is a #binary_tree #DFS_preorder problem.
|
|
|
|
|
|
|
|
Preorder, means root is at the "Pre" position, so the order is:
|
|
|
|
|
|
|
|
- Search root node
|
|
|
|
- Search left sub-tree
|
|
|
|
- Search right sub-tree
|
2022-07-04 15:08:23 +08:00
|
|
|
|
2022-07-04 15:01:29 +08:00
|
|
|
The recursion version is easy to implement.
|
|
|
|
|
2022-07-04 15:32:45 +08:00
|
|
|
Use Stacks for the iteration method, because stacks are FILO, the **subtree will get traversed first.**
|
|
|
|
And remember to push **right subtree** first, so that the left one will be traversed first.
|
|
|
|
|
2022-07-04 15:50:54 +08:00
|
|
|
The Morris traversal needs more understandings.
|
2022-07-04 15:32:45 +08:00
|
|
|
|
2022-07-04 15:01:29 +08:00
|
|
|
### Solution
|
2022-07-04 15:32:45 +08:00
|
|
|
Iteration
|
|
|
|
```cpp
|
|
|
|
/**
|
|
|
|
* Definition for a binary tree node.
|
|
|
|
* struct TreeNode {
|
|
|
|
* int val;
|
|
|
|
* TreeNode *left;
|
|
|
|
* TreeNode *right;
|
|
|
|
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
|
|
|
|
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
|
|
|
|
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left),
|
|
|
|
* right(right) {}
|
|
|
|
* };
|
|
|
|
*/
|
|
|
|
class Solution {
|
|
|
|
public:
|
|
|
|
vector<int> preorderTraversal(TreeNode *root) {
|
|
|
|
if (!root) {
|
|
|
|
return {};
|
|
|
|
}
|
|
|
|
|
|
|
|
// Using stacks, since FILO, the latest gets served first.
|
|
|
|
vector<int> answer;
|
|
|
|
stack<TreeNode *> pending;
|
|
|
|
|
|
|
|
do {
|
|
|
|
if (root != nullptr) {
|
|
|
|
answer.push_back(root->val);
|
|
|
|
// nothing gets pushed if is nullptr;
|
|
|
|
pending.push(root->right);
|
|
|
|
pending.push(root->left);
|
|
|
|
}
|
|
|
|
root = pending.top();
|
|
|
|
pending.pop();
|
|
|
|
} while (root || !pending.empty());
|
|
|
|
|
|
|
|
return answer;
|
|
|
|
}
|
|
|
|
};
|
|
|
|
|
|
|
|
```
|
2022-07-04 15:08:23 +08:00
|
|
|
Recursion, using private funcs:
|
|
|
|
```cpp
|
|
|
|
/**
|
|
|
|
* Definition for a binary tree node.
|
|
|
|
* struct TreeNode {
|
|
|
|
* int val;
|
|
|
|
* TreeNode *left;
|
|
|
|
* TreeNode *right;
|
|
|
|
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
|
|
|
|
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
|
|
|
|
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left),
|
|
|
|
* right(right) {}
|
|
|
|
* };
|
|
|
|
*/
|
|
|
|
class Solution {
|
|
|
|
public:
|
|
|
|
vector<int> preorderTraversal(TreeNode *root) {
|
|
|
|
// Use two functions:
|
|
|
|
vector<int> answer;
|
|
|
|
preorder(root, answer);
|
|
|
|
return answer;
|
|
|
|
}
|
|
|
|
|
|
|
|
private:
|
|
|
|
void preorder(TreeNode *root, vector<int> &answer) {
|
|
|
|
if (root == nullptr) {
|
|
|
|
return;
|
|
|
|
}
|
|
|
|
|
|
|
|
answer.push_back(root->val);
|
|
|
|
preorder(root->left, answer);
|
|
|
|
preorder(root->right, answer);
|
|
|
|
}
|
|
|
|
};
|
|
|
|
```
|
2022-07-04 15:01:29 +08:00
|
|
|
|
|
|
|
Recursion:
|
|
|
|
```cpp
|
|
|
|
/**
|
|
|
|
* Definition for a binary tree node.
|
|
|
|
* struct TreeNode {
|
|
|
|
* int val;
|
|
|
|
* TreeNode *left;
|
|
|
|
* TreeNode *right;
|
|
|
|
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
|
|
|
|
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
|
|
|
|
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left),
|
|
|
|
* right(right) {}
|
|
|
|
* };
|
|
|
|
*/
|
|
|
|
class Solution {
|
|
|
|
public:
|
|
|
|
vector<int> preorderTraversal(TreeNode *root) {
|
|
|
|
// If the node is empty
|
|
|
|
if (root == nullptr) {
|
|
|
|
return {};
|
|
|
|
}
|
|
|
|
// First check root
|
|
|
|
vector<int> answer;
|
|
|
|
|
|
|
|
answer.push_back(root->val);
|
|
|
|
|
|
|
|
vector<int> left = preorderTraversal(root->left);
|
|
|
|
answer.insert(answer.end(), left.begin(), left.end());
|
|
|
|
|
|
|
|
vector<int> right = preorderTraversal(root->right);
|
|
|
|
answer.insert(answer.end(), right.begin(), right.end());
|
|
|
|
|
|
|
|
return answer;
|
|
|
|
}
|
|
|
|
};
|
|
|
|
|
2022-07-04 15:08:23 +08:00
|
|
|
```
|