2022-09-02 15:50:18 +08:00
# Leetcode Rotate-Image
2022-09-02 15:40
> ##### Algorithms:
2022-09-03 15:41:36 +08:00
>
2022-09-02 15:50:18 +08:00
> #algorithm #math
2022-09-03 15:41:36 +08:00
>
2022-09-02 15:50:18 +08:00
> ##### Data structures:
2022-09-03 15:41:36 +08:00
>
> #DS #array
>
2022-09-02 15:50:18 +08:00
> ##### Difficulty:
2022-09-03 15:41:36 +08:00
>
2022-09-06 20:22:48 +08:00
> #coding_problem #difficulty_medium
2022-09-03 15:41:36 +08:00
>
2022-09-02 15:50:18 +08:00
> ##### Additional tags:
2022-09-03 15:41:36 +08:00
>
2022-09-02 15:50:18 +08:00
> #leetcode #CS_list_need_understanding
2022-09-03 15:41:36 +08:00
>
2022-09-02 15:50:18 +08:00
> ##### Revisions:
2022-09-03 15:41:36 +08:00
>
2022-09-02 15:50:18 +08:00
> N/A
##### Links:
2022-09-03 15:41:36 +08:00
2022-09-02 15:50:18 +08:00
- [Link to problem ](https://leetcode.com/problems/rotate-image/ )
- [Solution and explanation ](https://leetcode.com/problems/rotate-image/solution/ )
2022-09-03 15:41:36 +08:00
---
2022-09-02 15:50:18 +08:00
### Problem
You are given an `n x n` 2D `matrix` representing an image, rotate the image by **90** degrees (clockwise).
You have to rotate the image [**in-place** ](https://en.wikipedia.org/wiki/In-place_algorithm ), which means you have to modify the input 2D matrix directly. **DO NOT** allocate another 2D matrix and do the rotation.
#### Examples
**Example 1:**
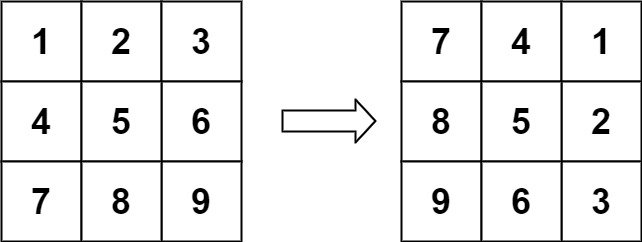
```
**Input:** matrix = [[1,2,3],[4,5,6],[7,8,9]]
**Output:** [[7,4,1],[8,5,2],[9,6,3]]
```
**Example 2:**
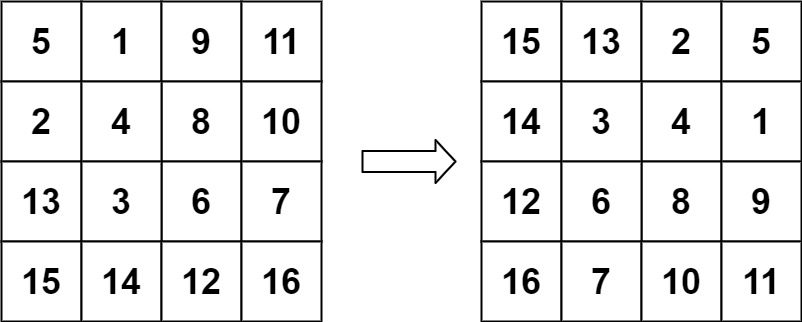
```
**Input:** matrix = [[5,1,9,11],[2,4,8,10],[13,3,6,7],[15,14,12,16]]
**Output:** [[15,13,2,5],[14,3,4,1],[12,6,8,9],[16,7,10,11]]
```
#### Constraints
### Thoughts
> [!summary]
> This is a #math problem.
Mainly two ways
#### Method 1: rotate in rings
2022-09-02 16:12:45 +08:00
#TODO complete in this method.
2022-09-02 15:50:18 +08:00
#### Method 2: transpose and reflect
2022-09-02 16:12:45 +08:00
transpose reflects the matrix in `y = x` ,
reflect changes matrix in axis y
2022-09-02 15:50:18 +08:00
### Solution
2022-09-02 16:12:45 +08:00
```cpp
class Solution {
void reflect(vector< vector < int > > & mat) {
// reflect in y axis.
int n = mat.size();
for (int i = 0; i < n ; i + + ) {
for (int j = 0, half = n / 2; j < half ; j + + ) {
swap(mat[i][j], mat[i][n - j - 1]);
}
}
}
void transpose(vector< vector < int > > & mat) {
int n = mat.size();
for (int i = 0; i < n ; i + + ) {
for (int j = 0; j < i ; j + + ) {
swap(mat[i][j], mat[j][i]);
}
}
}
public:
void rotate(vector< vector < int > > & matrix) {
// transpose and reflect
transpose(matrix);
reflect(matrix);
}
};
2022-09-03 15:41:36 +08:00
```