2022-09-03 15:06:04 +08:00
|
|
|
# Leetcode Search-A-2D-Matrix-II
|
|
|
|
|
|
|
|
2022-09-03 14:57
|
|
|
|
|
|
|
|
> ##### Algorithms:
|
2022-09-03 15:41:36 +08:00
|
|
|
>
|
2022-09-03 15:06:04 +08:00
|
|
|
> #algorithm #divide_and_conquer
|
2022-09-03 15:41:36 +08:00
|
|
|
>
|
2022-09-03 15:06:04 +08:00
|
|
|
> ##### Data structures:
|
2022-09-03 15:41:36 +08:00
|
|
|
>
|
|
|
|
> #DS #array
|
|
|
|
>
|
2022-09-03 15:06:04 +08:00
|
|
|
> ##### Difficulty:
|
2022-09-03 15:41:36 +08:00
|
|
|
>
|
2022-09-06 20:22:48 +08:00
|
|
|
> #coding_problem #difficulty_medium
|
2022-09-03 15:41:36 +08:00
|
|
|
>
|
2022-09-03 15:06:04 +08:00
|
|
|
> ##### Additional tags:
|
2022-09-03 15:41:36 +08:00
|
|
|
>
|
|
|
|
> #leetcode #CS_list_need_practicing
|
|
|
|
>
|
2022-09-03 15:06:04 +08:00
|
|
|
> ##### Revisions:
|
2022-09-03 15:41:36 +08:00
|
|
|
>
|
2022-09-03 15:06:04 +08:00
|
|
|
> N/A
|
|
|
|
|
|
|
|
##### Links:
|
2022-09-03 15:41:36 +08:00
|
|
|
|
2022-09-03 15:06:04 +08:00
|
|
|
- [Link to problem](https://leetcode.com/problems/search-a-2d-matrix-ii/)
|
2022-09-03 15:41:36 +08:00
|
|
|
|
|
|
|
---
|
|
|
|
|
2022-09-03 15:06:04 +08:00
|
|
|
### Problem
|
|
|
|
|
|
|
|
Write an efficient algorithm that searches for a value `target` in an `m x n` integer matrix `matrix`. This matrix has the following properties:
|
|
|
|
|
2022-09-03 15:41:36 +08:00
|
|
|
- Integers in each row are sorted in ascending from left to right.
|
|
|
|
- Integers in each column are sorted in ascending from top to bottom.
|
2022-09-03 15:06:04 +08:00
|
|
|
|
|
|
|
#### Examples
|
|
|
|
|
|
|
|
**Example 1:**
|
|
|
|
|
|
|
|
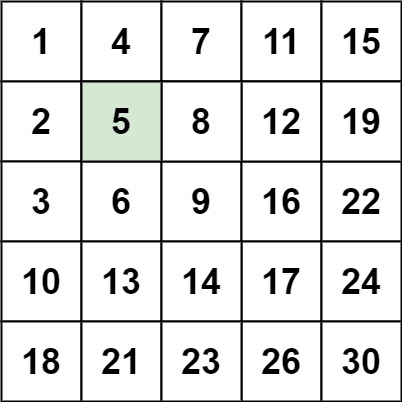
|
|
|
|
|
|
|
|
```
|
|
|
|
**Input:** matrix = [[1,4,7,11,15],[2,5,8,12,19],[3,6,9,16,22],[10,13,14,17,24],[18,21,23,26,30]], target = 5
|
|
|
|
**Output:** true
|
|
|
|
```
|
|
|
|
|
|
|
|
**Example 2:**
|
|
|
|
|
|
|
|
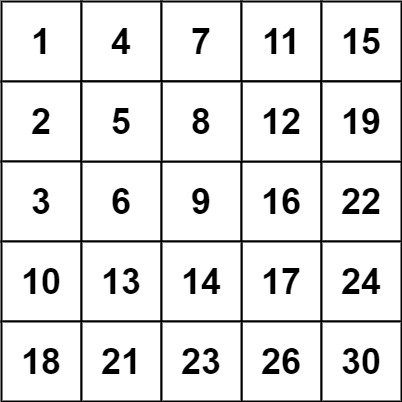
|
|
|
|
|
|
|
|
```
|
|
|
|
**Input:** matrix = [[1,4,7,11,15],[2,5,8,12,19],[3,6,9,16,22],[10,13,14,17,24],[18,21,23,26,30]], target = 20
|
|
|
|
**Output:** false
|
|
|
|
```
|
|
|
|
|
|
|
|
#### Constraints
|
|
|
|
|
2022-09-04 15:03:27 +08:00
|
|
|
- `m == matrix.length`
|
|
|
|
- `n == matrix[i].length`
|
|
|
|
- `1 <= n, m <= 300`
|
|
|
|
- `-109 <= matrix[i][j] <= 109`
|
|
|
|
- All the integers in each row are **sorted** in ascending order.
|
|
|
|
- All the integers in each column are **sorted** in ascending order.
|
|
|
|
- `-109 <= target <= 109`
|
2022-09-03 16:02:59 +08:00
|
|
|
|
2022-09-03 15:06:04 +08:00
|
|
|
### Thoughts
|
|
|
|
|
|
|
|
> [!summary]
|
|
|
|
> This is a #divide_and_conquer problem.
|
|
|
|
|
2022-09-03 15:41:36 +08:00
|
|
|
It's divide and conquer, because every time we do a action,
|
2022-09-03 15:06:04 +08:00
|
|
|
the problem is smaller.
|
|
|
|
|
|
|
|
Start from the top-right, (alternatively, bottom-left),
|
|
|
|
because walking left makes the number smaller, and down makes the number bigger.
|
|
|
|
|
|
|
|
### Solution
|
2022-09-03 15:41:36 +08:00
|
|
|
|
|
|
|
```cpp
|
|
|
|
class Solution {
|
|
|
|
public:
|
|
|
|
bool searchMatrix(vector<vector<int>> &matrix, int target) {
|
|
|
|
// search from top-right
|
|
|
|
int c = matrix[0].size() - 1;
|
|
|
|
int r = 0;
|
|
|
|
int m = matrix.size();
|
|
|
|
while (c >= 0 && r < m) {
|
|
|
|
if (matrix[r][c] > target) {
|
|
|
|
c--;
|
|
|
|
} else if (matrix[r][c] < target) {
|
|
|
|
r++;
|
|
|
|
} else {
|
|
|
|
return true;
|
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
return false;
|
|
|
|
}
|
|
|
|
};
|
2022-09-04 15:03:27 +08:00
|
|
|
```
|