2022-06-16 14:32:37 +08:00
# Leetcode Remove-Duplicates-From-Sorted-List
#### 2022-06-16 14:21
> ##### Data structures:
2022-09-03 15:41:36 +08:00
>
> #DS #linked_list
>
2022-06-16 14:32:37 +08:00
> ##### Difficulty:
2022-09-03 15:41:36 +08:00
>
2022-09-06 20:22:48 +08:00
> #coding_problem #difficulty_easy
2022-09-03 15:41:36 +08:00
>
2022-06-16 14:32:37 +08:00
> ##### Additional tags:
2022-09-03 15:41:36 +08:00
>
2022-06-16 14:32:37 +08:00
> #leetcode
2022-09-03 15:41:36 +08:00
>
2022-06-16 14:32:37 +08:00
> ##### Revisions:
2022-09-03 15:41:36 +08:00
>
2022-06-16 14:32:37 +08:00
> Initial encounter: 2022-06-16
2022-07-02 08:17:28 +08:00
> 1st. revision: 2022-07-02
2022-06-16 14:32:37 +08:00
##### Related topics:
2022-09-03 15:41:36 +08:00
2022-06-16 14:32:37 +08:00
##### Links:
2022-09-03 15:41:36 +08:00
2022-06-16 15:25:12 +08:00
- [Link to problem ](https://leetcode.com/problems/remove-duplicates-from-sorted-list/ )
2022-09-03 15:41:36 +08:00
---
2022-06-16 14:32:37 +08:00
### Problem
2022-09-03 15:41:36 +08:00
2022-06-16 15:25:12 +08:00
Given the `head` of a sorted linked list, _delete all duplicates such that each element appears only once_ . Return _the linked list **sorted** as well_ .
2022-06-16 14:32:37 +08:00
#### Examples
2022-09-03 15:41:36 +08:00
2022-06-16 15:25:12 +08:00
**Example 1:**
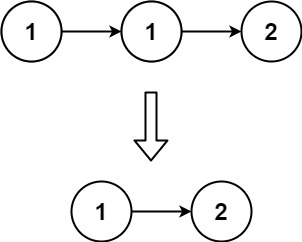
**Input:** head = [1,1,2]
**Output:** [1,2]
**Example 2:**
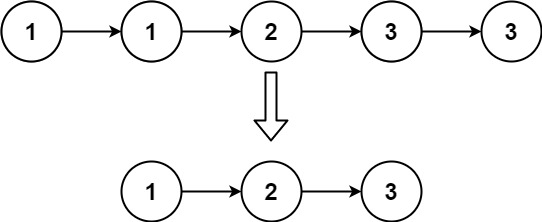
**Input:** head = [1,1,2,3,3]
**Output:** [1,2,3]
2022-06-16 14:32:37 +08:00
#### Constraints
2022-09-03 15:41:36 +08:00
- The number of nodes in the list is in the range `[0, 300]` .
- `-100 <= Node.val <= 100`
- The list is guaranteed to be **sorted** in ascending order.
2022-06-16 14:32:37 +08:00
### Thoughts
This can be implemented using recursion and iteration, like [[Leetcode Reverse-Linked-List]].
Recursion is not recommended, since it will take up O(n) space.
In iteration, one pointer or 2 pointers can be used.
To understand the ptr->next->next = ptr->next, see[[Leetcode Reverse-Linked-List#Solution]]
### Solution
One pointer method
2022-09-03 15:41:36 +08:00
2022-06-16 14:32:37 +08:00
```cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode *deleteDuplicates(ListNode *head) {
ListNode *ptr = head;
while (ptr != NULL & & ptr->next != NULL) {
if (ptr->val == ptr->next->val) {
// Duplicate found
auto tmp = ptr->next;
ptr->next = ptr->next->next;
delete tmp;
// I done set ptr to ptr->next, in case new ptr->next is duplicated
} else {
ptr = ptr->next;
}
}
return head;
}
};
```